어제 구조 설계와 header, footer 작업을 완료해서 오늘은 서브페이지들을 작업하려 한다.
1) home visual
<div className="main_slider">
<Swiper
modules={[Navigation, Scrollbar, A11y, Autoplay]}
spaceBetween={0}
slidesPerView={1}
navigation
loop={true}
pagination={{ clickable: true }}
scrollbar={{ draggable: true }}
autoplay={true}
onSwiper={(swiper) => console.log(swiper)}
onSlideChange={() => setActive(!active)}
>
<SwiperSlide id={onAni}><img src="./img/main/main_slider_1.jpg" alt="" /></SwiperSlide>
<SwiperSlide id={onAni}><img src="./img/main/main_slider_2.jpg" alt="" /></SwiperSlide>
<SwiperSlide id={onAni}><img src="./img/main/main_slider_3.jpg" alt="" /></SwiperSlide>
</Swiper>
</div>
어제 header 부분에 사용했던 swiper를 똑같이 적용시켰다.
swiperslide에 붙은 id는 animation을 적용시키기 위해 넣었다.
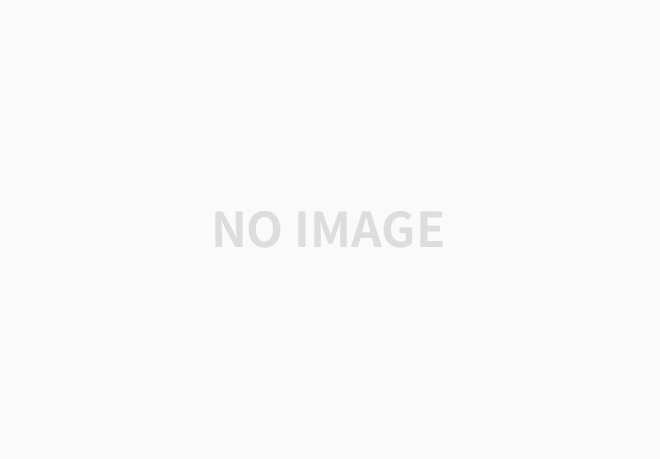
swiper가 진행될 때마다 class의 이름이 변경되는데 이 부분과 slide가 될 때마다 실행되는 함수를 이용해 ani id를 붙여주었다.
onSlideChange={() => setActive(!active)}
const onAni = 'ani'
const [active, setActive] = useState(true)
useEffect(() => {
const onAni = 'ani'
}, [active])
#ani {
animation: mainSlider 10s;
}
}
@keyframes mainSlider {
from {
transform: scale(1)
}
to {
transform: scale(1.2);
}
}
2) noticeList
어제 작성했던 공통부분을 제외한 게시판과 글 작업을 했다.
export default [
{
id: 1,
title: '픽업앤충전 서비스 런칭 이벤트 안내',
date: '2021.05.12',
view: '287',
images: [
{ img: './img/noti/20210512152052_58344753.png' },
{ img: './img/noti/20210512152055_34496955.png' },
{ img: './img/noti/20210512152058_65778382.png' },
{ img: './img/noti/20210512152101_73472584.png' },
],
txt: `현대자동차 전기차 이용고객을 위해\n충전을 대신해서 가져다 드리는 픽업앤충전서비스가 런칭했습니다!\n\n픽업앤충전서비스 런칭 이벤트로\n무상 쿠폰 지급이 되고 있다고 하니\n마이현대App 쿠폰함에서 확인해보세요~!\n\n현재 제주도에서 제공되는 서비스는 아니지만,\n서울 거주자이면서 제주도에서 렌트를 하고 계신 분이라면\n잊지말고 꼭 무료로 서비스를 받으세요~\n`
},
.
.
.
제일 먼저 data를 파싱해 js파일로 만들었다.
const Noticelist = () => {
return (
<ContainerNotice>
<div id="container">
<div className="inner">
<div className="notice_list">
<table>
<caption>NO, 제목, 작성일, 조회수에 대한 내용</caption>
<colgroup>
<col style={{ width: '13%' }} />
<col />
<col style={{ width: '13%' }} />
<col style={{ width: '13%' }} />
</colgroup>
<thead>
<tr>
<th>NO</th>
<th>제목</th>
<th>작성일</th>
<th>조회수</th>
</tr>
</thead>
<tbody id="board_list">
{
noticeData.slice().reverse().map(item =>
<tr key={item.id}>
<td>{item.id}</td>
<td className='subject'>
<strong><Link to={`/noticeList/${item.id}`}>{item.title}</Link></strong></td>
<td>{item.date}</td>
<td>{item.view}</td>
</tr>
)
}
</tbody>
</table>
</div>
<div className="paginate" id="board_paging">
<a className='btn_prev_2 disable' href=""></a>
<a className='btn_prev disable' href=""></a>
<ol>
<li><a href="" className='on'>1</a></li>
</ol>
<a className='btn_next disable' href=""></a>
<a className='btn_next_2 disable' href=""></a>
</div>
</div>
</div>
</ContainerNotice>
);
};
thead 부분은 공통 사항이므로 작성을 했고 tbody는 게시글 수만큼 출력되어야 하므로 map을 사용해 게시판이 만들어질 수 있도록 했다.
<strong><Link to={`/noticeList/${item.id}`}>{item.title}</Link></strong></td>
마지막으로 게시판 제목 부분에 Link를 추가해 해당 게시글의 id를 주소 번호로 적용되도록 했다.
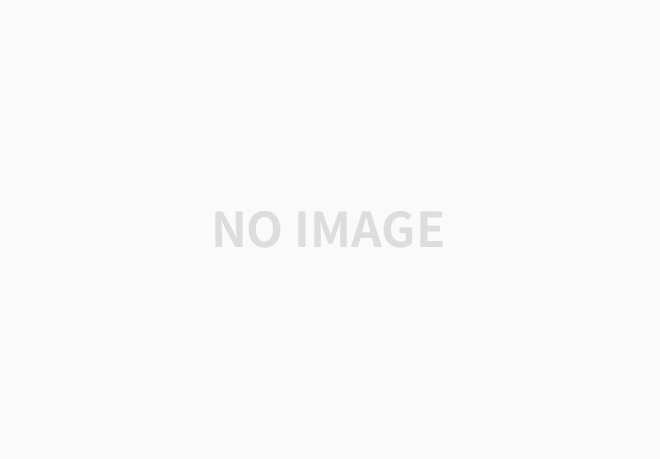
3) noticedetail
const currentItem = noticeData.find(item => item.id === parseInt(id));
if (!currentItem) {
return <p className='notfind' style={{ textAlign: 'center', margin: 200, fontSize: 50 }}>해당 공지사항을 찾을 수 없습니다.</p>;
}
const { title, date, txt, images } = currentItem
게시판의 제목을 누르면 id를 가져와 게시판 data에서 find를 사용해 해당 데이터만 가져오게 했고, 만약 id를 잘못 가져오거나 다른 번호의 주소가 들어가게 되면 '해당 공지사항을 찾을 수 없습니다.'라는 메시지가 출력된다.
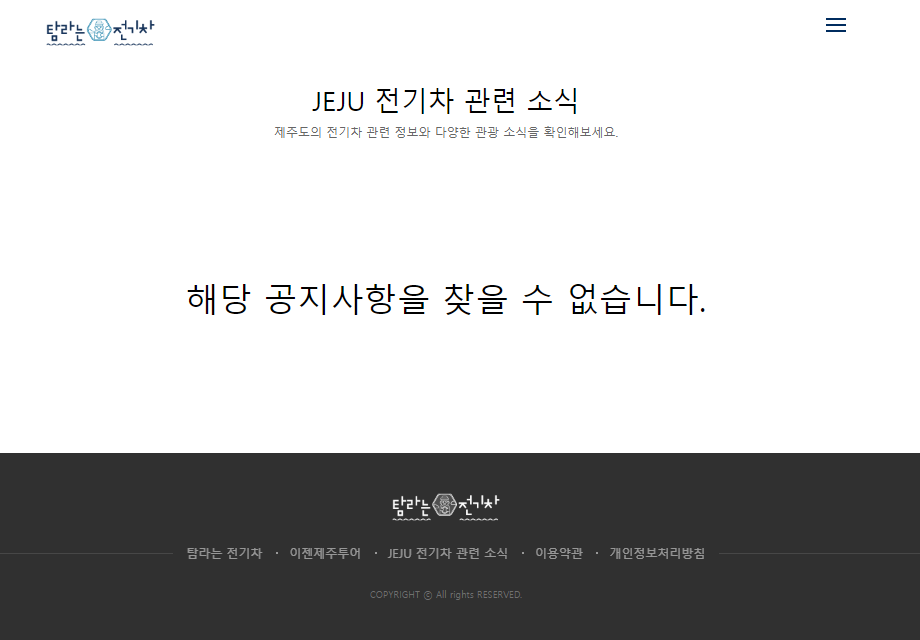
<div className="paginate">
<Link className="btn_prev" to={Number(id) === 1 ? `/noticeList/${Number(id)}` : `/noticeList/${Number(id) - 1}`} >이전글</Link>
<Link to="/noticeList" className="btn_list">목록보기</Link>
<Link className="btn_next" to={Number(id) === noticeData.length ? `/noticeList/${Number(id)}` : `/noticeList/${Number(id) + 1}`} >다음글</Link>
</div>
목록보기 버튼은 noticeList로 이동하게 Link를 사용했고, 이전글, 다음글 버튼은 현재 보고 있는 게시글의 id에서 1이 증감된 페이지로 이동하게 했다.
만약 첫 게시글이거나 마지막 게시글인 경우 이동이 되지 않도록 삼항연산자를 넣었다.
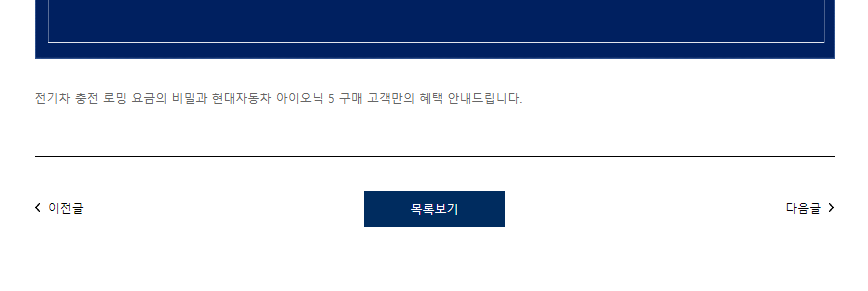